우선 위경도 표현에는 두 가지 방식이 있다..
- 소수점표현(DD,Decimal Degree) : 예) 37.397
- 도분초 표현(DMS, Degree Minutes Seconds) 예) 37º 23' 49.2"
소수점 표현같은 경우에는 구글지도에서 쉽게 확인 가능..
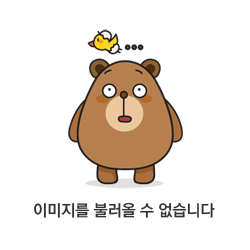
1. 소수점 표현 방식 -> DMS 표현방식 변환
예) 37.397 을 DMS 표현으로 변환
- 도 : 소수점 좌표 값의 정수(37)
- 분 : 37을 제외한 0.397 X 60 = 23.82 에서 소수점 앞의 정수(23)
- 초 : 0.82 X 60 = 49.2 에서 소수점 포함 앞의 4자리(49.2)
=> 37º 23' 49.2"
//소수점 표현 위도,경도를 DMS 표현으로 변환
public HashMap<String ,Object> getDmsByLatLon(Object data) throws Exception{
//data = 37.397
HashMap<String,Object> result = new HashMap<>();
try {
String[] dataArray = data.toString().split("\\.");
//도 : 소수점 좌표 값의 정수
String dataDegree = dataArray[0];
String dataMinutesFull = String.valueOf(Double.parseDouble("0." + dataArray[1]) * 60);
//분
String dataMinutes = dataMinutesFull.split("\\.")[0];
//초
String dataSeconds;
if(String.valueOf(Double.parseDouble("0." + dataMinutesFull.split("\\.")[1]) * 60).length()<5){
dataSeconds = String.valueOf(Double.parseDouble("0." + dataMinutesFull.split("\\.")[1]) * 60);
}else {
dataSeconds = String.valueOf(Double.parseDouble("0." + dataMinutesFull.split("\\.")[1]) * 60).substring(0, 5);
}
result.put("degree", dataDegree);
result.put("minutes", dataMinutes);
result.put("seconds", dataSeconds);
}catch(Exception e){
e.printStackTrace();
}
return result;
}
//result = {seconds=49.19, minutes=23, degree=37}
2. DMS 표현 방식 -> 소수점 표현 방식 변환
예) 37º 23' 49.2" 를 DD 표현으로 변환하기
식은 다음과 같다..
도 + ( 분 + (초 / 60 ) ) / 60
37 + ( 23 + (49.2/60) ) /60 = 37.397
double latDD = MaxDeg + (latMin + latSec / 60) / 60;
3. 두 좌표간의 거리
두 좌표간의 거리를 구하려면 두 좌표는 DMS표현 방식이어야 함..
도는 도, 분은 분, 초는 초끼리 연산을 한다.
37° 33′ 58.97″ N , 126° 58′ 39.78″ E (서울시청)
- 37° 33′ 56.08″ N , 126° 58′ 41.10″ E (서울광장)
= 0 ° 0 ′ 2.89" , 0 ° 0 ′ -1.32"
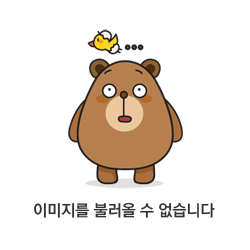
//지리좌표간 두 점의 거리 계산을 위한 DMS연산
public HashMap<String, Double> DMSCalculationForDistance(Double targetDeg, Double targetMin, Double targetSec, Double opDeg, Double opMin, Double opSec)throws Exception{
HashMap<String, Double> result = new HashMap<>();
result.put("resultDeg",targetDeg-opDeg);
result.put("resultMin",targetMin-opMin);
result.put("resultSec",targetSec-opSec);
return result;
}
//DMS 연산 결과로 거리 구하기
public Double getDistanceByDms(Double latDeg,Double latMin, Double latSec,Double lonDeg, Double lonMin, Double lonSec) throws Exception{
Double result;
result = Math.sqrt(Math.pow(latDeg*88.9036+latMin*1.4817+latSec*0.0246,2) + Math.pow(lonDeg*111.3194+lonMin*1.8553+lonSec*0.0309,2));
return result;
}
4. 특정지점에서 특정 거리의 좌표 구하기
이 경우도 DMS 표현방식의 좌표로 계산하였다.
예를 들어 37° 33′ 58.97″ , 126° 58′ 39.78″ 좌표에서 서쪽으로 5km 떨어진 지점의 좌표를 구한다거나 하는 경우,
5km 만큼의 경도 수치를 구해서 빼준다.
동쪽으로 5km 떨어진 지점이라면 수치를 더해주고, 북쪽으로 5km 떨어진 지점이라면 5km만큼의 위도 수치를 더해준다.
위경도 1분, 1초 값은 아래 수치로 계산하였다.
- 위도 1분 = 1.85km, 1초 = 30.8m
- 경도 1분 = 1.48km, 1초 = 25m
//위도 5km = 약 2분 42.2초(1분=1.85km, 1초=30.8m로 계산)
int latDistanceMin = 2;
Double latDistanceSec = 42.2D;
//경도 5km = 약 3분 22.4초(1분=1.48km, 1초=25m로 계산)
int lonDistanceMin = 3;
Double lonDistanceSec = 22.4D;
//DMS 표현 위경도에 특정 거리를 더하는 연산
public HashMap<String,Double> latlonCalDistance (Double targetDeg, Double targetMin, Double targetSec, Double opDeg, Double opMin, Double opSec,String operator) throws Exception{
HashMap<String,Double> result = new HashMap<>();
Double resultSec;
Double resultMin;
Double resultDeg;
//더하기 연산
if(operator =="+"){
if(targetSec+opSec>=60){
resultSec = targetSec+opSec-60;
resultMin = targetMin+opMin+1;
if(resultMin >=60){
resultMin = resultMin -60;
resultDeg = targetDeg + opDeg + 1;
}else{
resultDeg = targetDeg + opDeg;
}
}else{
resultSec = targetSec+opSec;
resultMin = targetMin+opMin;
if(resultMin >=60){
resultMin = resultMin -60;
resultDeg = targetDeg + opDeg + 1;
}else{
resultDeg = targetDeg +opDeg;
}
}
//빼기 연산
}else{
if(opSec > targetSec){
resultSec = 60-opSec+targetSec;
resultMin = targetMin-1;
if(opMin > targetMin){
resultMin = 60-opMin+resultMin;
resultDeg = targetDeg -1 - opDeg;
}else{
resultMin = resultMin-opMin;
resultDeg = targetDeg - opDeg;
}
}else{
resultSec = targetSec - opSec;
if(opMin > targetMin){
resultMin = 60-opMin+targetMin;
resultDeg = targetDeg -1 - opDeg;
}else{
resultMin = targetMin-opMin;
resultDeg = targetDeg - opDeg;
}
}
}
result.put("resultDeg",resultDeg);
result.put("resultMin",resultMin);
result.put("resultSec",resultSec);
return result;
}
참고 링크
위도 경도 계산법
위도 경도를 아래 공식에 대입해서 d(거리)값을 구한 상태 입니다. ==========점(x1, y1)과 직선(ax+by+c=0) 사이의 거리(d) 구하는 공식 ====================== d = |ax1 + by1 + c | / sqrt(a^2 + b^2) =======..
lovestudycom.tistory.com
지리좌표 거리 - 위키백과, 우리 모두의 백과사전
지리좌표 거리(Geographical distance)는 경위도좌표계를 기반으로 하는 좌표체계에서 얻어지는 두 지점의 좌표로 부터 측정되는 거리측량을 가리킨다. GPS로 부터 좌표값을 얻어 두 지점간의 지리좌
ko.wikipedia.org