JavaScript에서 AJAX 나 Fetch로 JSON 데이터 전송, SpringBoot의 Controller에서 데이터 받기
AJAX로 데이터 전송하기 : Content -Type 지정하지 않은 경우
1) javascript
Content Type을 명시하지 않으면 기본 타입은 application/x-www-form-urlencoded 으로 전송된다.
let obj={
"fromYear" : $('#fromYear').val(),
"fromMonth" : $('#fromMonth').val(),
"fromDay" : $('#fromDay').val(),
"toYear" : $('#toYear').val(),
"toMonth" : $('#toMonth').val(),
"toDay" : $('#toDay').val()
};
$.ajax({
type: "post",
url : "http://localhost:8080/test2",
data : obj,
success : function (data){
},
})
2) 전송되는 데이터 확인
요청 헤더의 Content-Type 은 application/x-www-form-urlencoded; charset=UTF-8 이며
body의 데이터가 FormData로 표시된다.
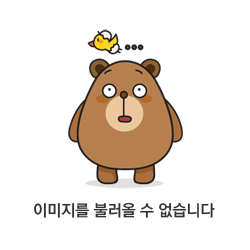
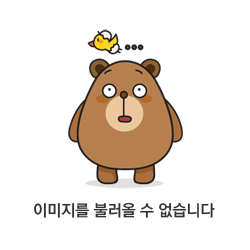
3) 데이터 수신 Controller
파라미터는 변수명이나 VO로 받는다.
@RequestMapping(value = "/test2", method = RequestMethod.POST)
@ResponseBody
public void test2(String fromYear, String fromMonth, String fromDay,String toYear, String toMonth, String toDay){
System.out.println(fromYear+fromMonth+fromDay);
//2021825
}
혹은 HttpServletRequest로 받는다.
HttpServletRequest로 받는 경우 데이터를 좀 가공함.
@RequestMapping(value = "/test2", method = RequestMethod.POST)
@ResponseBody
public ResponseEntity<Resource> test(HttpServletRequest req)throws IOException{
//readBody 메소드 호출
String body = readBody(req);
System.out.println(readBody(req));
//fromYear=2021&fromMonth=8&fromDay=25&toYear=2021&toMonth=8&toDay=25
//String 으로 바꾼 body부를 JSON형태로 변환하여 사용
org.json.simple.parser.JSONParser parser = new org.json.simple.parser.JSONParser();
Object obj = parser.parse(body);
JSONObject jsonObj = (JSONObject) obj;
String fromYear = (String) jsonObj.get("fromYear");
return new ResponseEntity<>("성공", HttpStatus.OK);
}
//body 읽는 메소드
public static String readBody(HttpServletRequest request) throws IOException {
BufferedReader input = new BufferedReader(new InputStreamReader(request.getInputStream()));
StringBuilder builder = new StringBuilder();
String buffer;
while ((buffer = input.readLine()) != null) {
if (builder.length() > 0) {
builder.append("\n");
}
builder.append(buffer);
}
return builder.toString();
}
AJAX로 데이터 전송하기 : Content -Type 을 JSON으로 보내는 경우
1) javascript
보낼 데이터를 JSON.stringify()로 감싸주어야 한다.
$.ajax({
type: "post",
url : "http://localhost:8080/test2",
headers: {'Content-Type': 'application/json'},
///보낼 데이터를 JSON.stringify()로 감싸주어야 함
data : JSON.stringify(obj),
success : function (data){
console.log(data);
},
error : function(e){
}
})
2) 전송되는 데이터 확인
요청 헤더의 Content-Type은 application/json이며
body의 데이터가 Request Payload로 표시된다
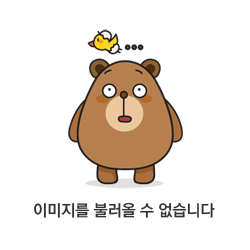

3) 데이터 수신 Controller
파라미터를 HttpServletRequest로 받는다.
@RequestMapping(value = "/test", method = RequestMethod.POST)
@ResponseBody
public ResponseEntity<Resource> test(HttpServletRequest req)throws IOException{
System.out.println(readBody(req));
//{"fromYear":"2021","fromMonth":"8","fromDay":"25","toYear":"2021","toMonth":"8","toDay":"25"}
}
public static String readBody(HttpServletRequest request) throws IOException {
BufferedReader input = new BufferedReader(new InputStreamReader(request.getInputStream()));
StringBuilder builder = new StringBuilder();
String buffer;
while ((buffer = input.readLine()) != null) {
if (builder.length() > 0) {
builder.append("\n");
}
builder.append(buffer);
}
return builder.toString();
}
fetch로 데이터 전송하기
1) javascript
fetch("http://localhost:8080/test",{
method : 'POST',
mode : 'cors',
cache : 'no-cache',
//Content Type은 json으로 명시한다.
headers: {'Content-Type': 'application/json'},
credentials : 'same-origin',
redirect : 'follow',
referrer : 'no-referrer',
body: JSON.stringify(obj),
}).then(response => console.log(response));
2) 전송되는 데이터 확인
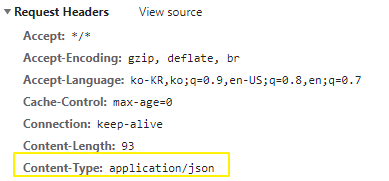
request payload로 전송이됨

3) 데이터 수신 Controller
파라미터는 HttpServletRequest로 받는다.
@RequestMapping(value = "/test", method = RequestMethod.POST)
@ResponseBody
public ResponseEntity<Resource> test(HttpServletRequest req)throws IOException{
System.out.println(readBody(req));
//{"fromYear":"2021","fromMonth":"8","fromDay":"25","toYear":"2021","toMonth":"8","toDay":"25"}
}
public static String readBody(HttpServletRequest request) throws IOException {
BufferedReader input = new BufferedReader(new InputStreamReader(request.getInputStream()));
StringBuilder builder = new StringBuilder();
String buffer;
while ((buffer = input.readLine()) != null) {
if (builder.length() > 0) {
builder.append("\n");
}
builder.append(buffer);
}
return builder.toString();
}
참고
What's the difference between "Request Payload" vs "Form Data" as seen in Chrome dev tools Network tab
I have an old web application I have to support (which I did not write). When I fill out a form and submit then check the "Network" tab in Chrome I see "Request Payload" where I would normally see...
stackoverflow.com
'코딩 관련 > Javascript와 jQuery, JSON' 카테고리의 다른 글
[JavaScript] fetch, then 사용 (0) | 2021.09.10 |
---|---|
[AJAX] ajax success, error 사용 / error 파라미터 (5) | 2021.08.31 |
[JavaScript] 엑셀 파일 읽기. read excel file (2) | 2021.08.19 |
[JavaScript] datepicker (0) | 2021.08.12 |
[Javascritpt] 로컬 텍스트 파일 읽기 (1) | 2021.07.26 |